Let’s begin with a simple Java program that displays the message Hello World! on the
console by using Netbeans IDE.
Step 1: After opening Netbeans IDE we select File -> New Project
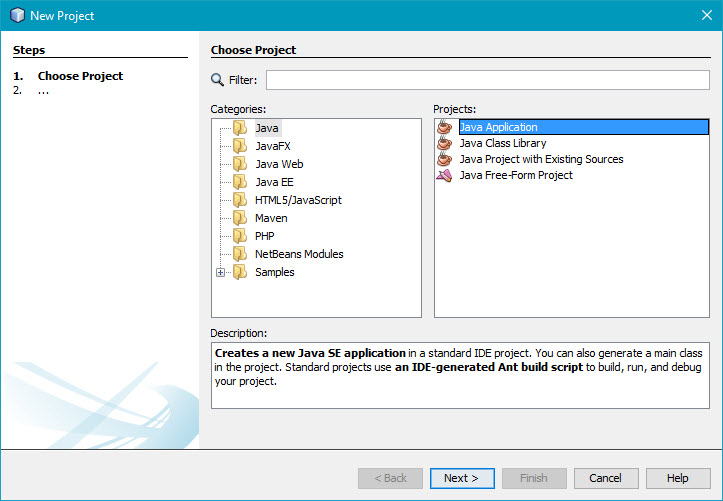
Step 2: In the next dialog, we type Project Name and select Project Location for the application
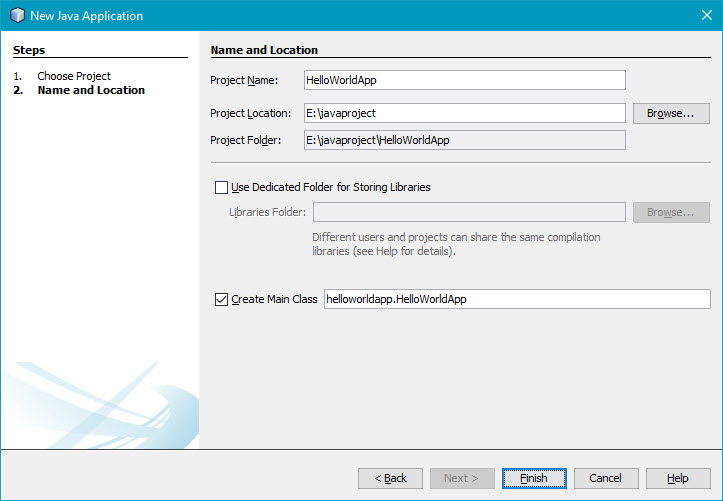
Step 3: Pass the following code into the editor and click Run
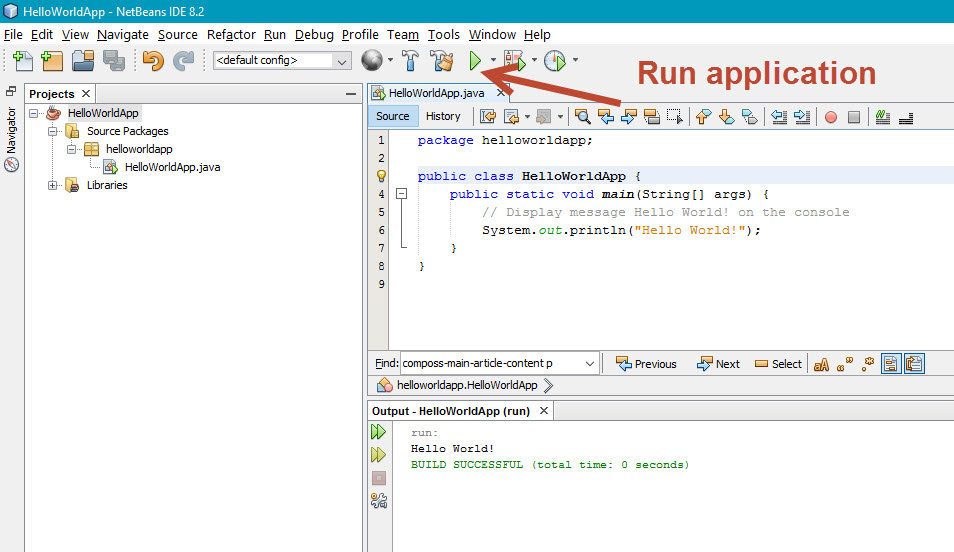
public class HelloWorldApp { public static void main(String[] args) { // Display message Hello World! on the console System.out.println("Hello World!"); } }
Line 1 defines a class. Every Java program must have at least one class. Each class has a name. By convention, class names start with an uppercase letter. In this example, the class name is HelloWorldApp.
Line 2 defines the main method. The program is executed from the main method. A class may contain several methods. The main method is the entry point where the program begins execution. A method is a construct that contains statements. The main method in this program contains the System.out.println statement. This statement displays the string Hello World! on the console (line 4).
String is a programming term meaning a sequence of characters. A string must be enclosed in double quotation marks. Every statement in Java ends with a semicolon (;), known as the statement terminator.
Keywords have a specific meaning to the compiler and cannot be used for other purposes in the program. For example, when the compiler sees the word class, it understands that the word after class is the name of the class. Other keywords in this program are public, static, and void.
Line 3 is a comment that documents what the program is and how it is constructed. Comments help programmers to communicate and understand the program. They are not programming statements and thus are ignored by the compiler. In Java, comments are preceded by two slashes (//) on a line, called a line comment, or enclosed between /* and */ on one or several lines, called a block comment or paragraph comment. When the compiler sees //, it ignores all text after // on the same line. When it sees /*, it scans for the next */ and ignores any text
between /* and */.
Here are examples of comments:
// This application program displays Welcome to Java! /* This application program displays Welcome to Java! */ /* This application program displays Welcome to Java! */