When reduced to their most basic form, all computer decisions are yes-or-no decisions. That is, the answer to every computer question is yes or no (or true or false, or on or off). This is because computer circuitry consists of millions of tiny switches that are either on or off, and the result of every decision sets one of these switches in memory.
boolean Data Type
How do you compare two values, such as whether a distance is greater than 0, equal to 0, or less than 0? Java provides six relational operators (also known as comparison operators), shown in the following table, which can be used to compare two values:
Operator | Description | Example (a=10, b=15) | Result |
---|---|---|---|
== | Equality operator | a==b | false |
!= | Not Equal to operator | a!=b | true |
> | Greater than | a>b | false |
< | Less than | a<b | true |
>= | Greater than or equal to | a>=b | false |
<= | Less than or equal to | a<=b | true |
The result of the comparison is a Boolean value: true or false. For example, the following statement displays true:
int distance = 100; System.out.println(distance&amp;amp;amp;amp;gt;=100); //print true
if and if…else Statements
In Java, when you want to take an action if a Boolean expression is true, you use an if statement. If you want to take an action when a Boolean expression is true but take a different action when the expression is false, you use an if else statement. You will understand about if else statement clearly via statement:
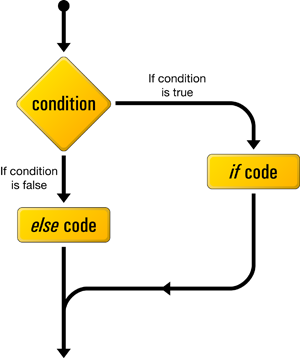
Syntax:
if(condition) statement; or if (condition) statement1; else statement2;
Example
public class Test { public static void main(String args[]) { int x = 2; if( x &amp;amp;amp;amp;lt; 10 ) { System.out.print("Expression is true"); }else { System.out.print("Expression is false"); } } }
Using the switch Statement
By nesting a series of if and else statements, you can choose from any number of alternatives. For example, suppose you want to display a student’s class year based on a stored number.
int year = 2; if(year == 1) System.out.println("Freshman"); else if(year == 2) System.out.println("Sophomore"); else if(year == 3) System.out.println("Junior"); else if(year == 4) System.out.println("Senior"); else System.out.println("Invalid year");
An alternative to using the series of nested if statements shown in above example is to use the switch statement. The switch statement is useful when you need to test a single variable against a series of exact integer (including int, byte, and short types), character, or string values.
The switch statement uses four keywords:
- switch starts the statement and is followed immediately by a test expression enclosed in parentheses.
- case is followed by one of the possible values for the test expression and a colon.
- break optionally terminates a switch statement at the end of each case.
- default optionally is used prior to any action that should occur if the test variable does not match any case.
int year =2; switch (year) { case 1: System.out.println("Freshman"); break; case 2: System.out.println("Sophomore"); break; case 3: System.out.println("Junior"); break; case 4: System.out.println("Senior"); break; default: System.out.println("Invalid year"); }
The switch statement shown in the above example begins by evaluating the year variable shown in the first line. For example, if year is equal to 3, the statement that displays “Junior” executes. The break statement bypasses the rest of the switch statement, and execution continues with any statement after the closing curly brace of the “switch” statement. If the year variable does not contain the same value as any of the case statements, the default statement is executed.
You can leave out the break statements in a switch statement. However, if you omit the break and the program finds a match for the test variable, all the statements within the switch statement execute from that point forward. For example, if you omit each break statement in the code, when the year is 3, the first two cases are bypassed, but Junior, Senior, and Invalid year all are output. You should intentionally omit the break statements if you want all subsequent cases to execute after the test variable is matched.