There are many ways to create a Spring Boot application. The simplest way is to use Spring Initializr at
http://start.spring.io/, which is an online Spring Boot application generator. In this post, you will learn
how to create a simple Spring Boot web application serving a simple HTML page and explore various aspects
of a typical Spring Boot application.
Using Spring Initializr
You can point your browser to http://start.spring.io/ and see the project details
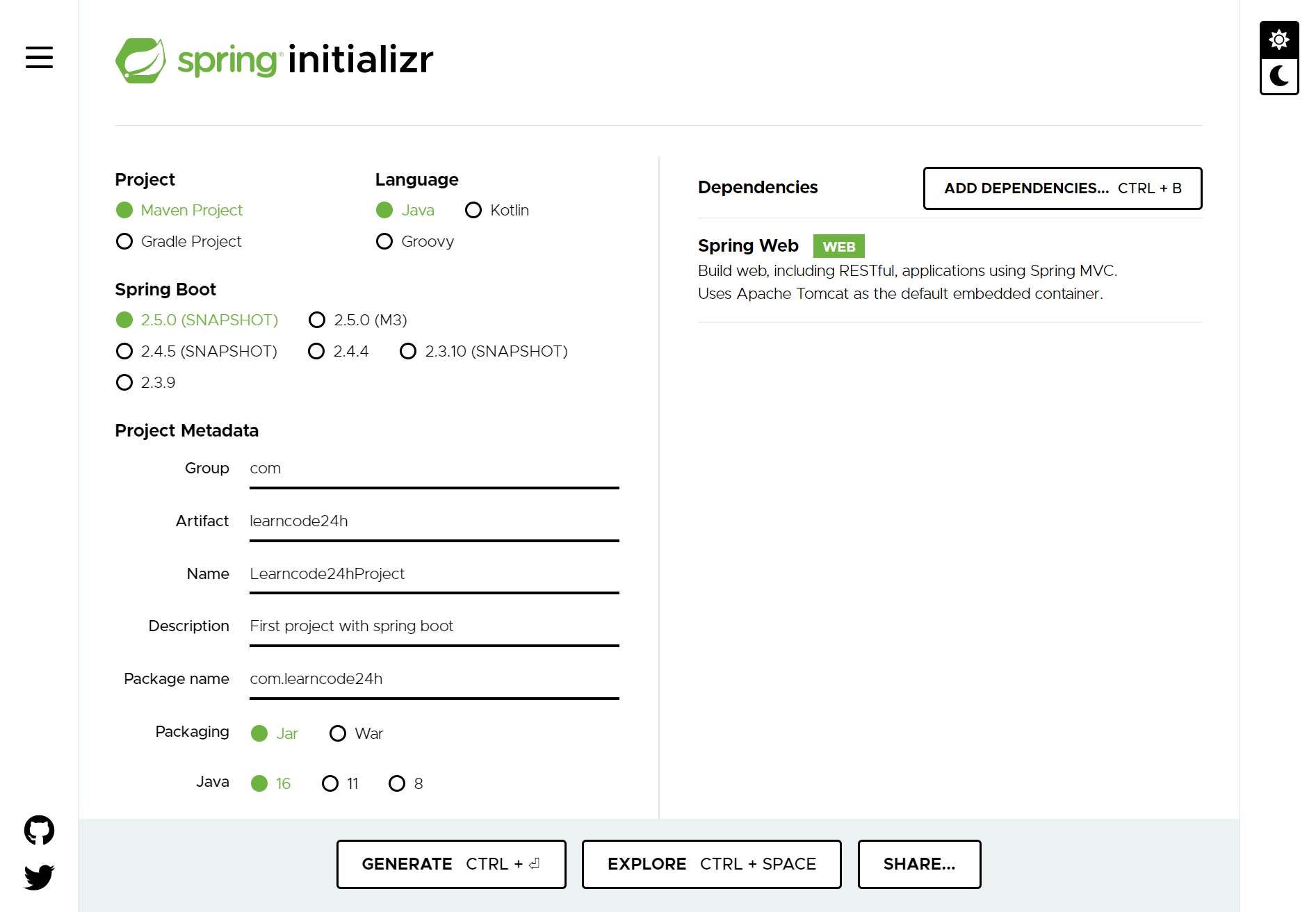
- Select Maven Project and Spring Boot version
- Enter the Maven project details as follows:
- Group: com
- Artifact: learncode24h
- Name: Learncode24hProject
- Package Name: com.learncode24h
- Packaging: JAR
- Java version: 16
- Language: Java
In dependencies, you will see many starter modules organized into various categories, like Core, Web, Data… In this tutorial I’ll select the Web.
Click on the Generate button and import it to your IDE.
IDE For Spring Boot
Spring tools for Eclipse
To make it even easier to write modern Spring Boot applications, the latest generation of the Spring Tools for the Eclipse IDE are well suited for getting started with Spring Boot and working on large microservice applications that are based on Spring Boot. This article walks you through the most important features of the tooling and provides great insight into a number of tips and tricks along the way.
Intellij IDEA
Intellij IDEA is a powerful commercial IDE with great features, including support for Spring Boot.
Spring framework support comes only with the commercial intellij idea Ultimate edition, not with the Community edition, which is free. if you want to use the intellij idea Community edition, you can generate the project using Spring initializr and import it into intellij idea as a Maven/Gradle project.
NetBeans IDE
This IDE provides built-in support for Spring Framework 4.x and 3.x. Framework libraries are packaged with the IDE and are automatically added to the project classpath when the framework is selected. Configuration settings are provided, such as naming and mapping of the Spring Web MVC DispatcherServlet
. The JSTL library can optionally be registered upon project creation. Support for Spring XML bean configuration files is also provided, including the following functionality:
- Code completion. Invoked in Spring XML configuration files for Java classes as well as bean references.
- Navigation. Hyperlinking of Java classes and properties mentioned in Spring bean definitions, as well as hyperlinking to other Spring bean references.
- Refactoring. Renaming of references to Java classes in Spring XML configuration files.
We’ll review the generated code from Spring Inittializr
package com.learncode24h.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController{
@RequestMapping("/")
public String home(Model model) {
return "index.html";
}
}
This is a simple SpringMVC controller with one request handler method for URL /, which returns the view named index.html. View name ‘index.html’ is located at src/main/public.
Comments are closed.